Lazy loading is a design pattern that delays the loading of non-essential resources until they are needed. This technique improves performance and enhances user experience by reducing initial load time.
Lazy loading optimizes web performance by loading images, videos, and other elements only when they become visible on the user’s screen. It significantly enhances page speed, making websites more efficient and user-friendly. By minimizing the initial amount of data loaded, lazy loading reduces bandwidth usage, which is crucial for mobile users.
This technique is especially beneficial for content-heavy sites, such as blogs and e-commerce platforms. Implementing lazy loading can lead to improved search engine rankings, as faster-loading pages contribute to better user engagement. Embracing this method can ultimately drive more traffic and increase conversions.
Introduction To Lazy Loading
Lazy loading is a technique for improving website performance. It delays loading images and other resources until they are needed. This speeds up the initial loading time of a webpage. Users see content faster, enhancing their experience.
The Basics Of Website Performance
Website performance is crucial for user satisfaction. A slow site can frustrate visitors and lead to high bounce rates. Here are some key factors that affect performance:
- Load Time: The time it takes for a page to fully load.
- Interactivity: How quickly users can interact with elements.
- Visual Stability: The avoidance of layout shifts during loading.
Improving these factors can keep users engaged. Lazy loading is one effective way to boost performance.
Lazy Loading Essentials
Lazy loading focuses on loading content only when needed. This method uses JavaScript to identify when an element comes into view. Benefits include:
- Reduced initial load time
- Lower server bandwidth usage
- Better user experience
Here’s how lazy loading works:
- Images or videos are marked for lazy loading.
- They load as the user scrolls down the page.
- This keeps the initial content light and fast.
Implementing lazy loading can be simple. Use the loading="lazy"
attribute in your HTML images:
This single line can significantly improve performance. Lazy loading is essential for modern web design.
The Mechanics Of Lazy Loading
Lazy loading is a technique that improves web performance. It helps load content only when needed. This method can boost speed and reduce data usage. Understanding how lazy loading operates is essential for developers and website owners.
How Lazy Loading Operates
Lazy loading works by delaying the loading of images and other resources. Here’s how it typically operates:
- The user opens a webpage.
- Only the visible content loads initially.
- As the user scrolls, additional content loads.
- This process continues until all content is visible.
This approach saves bandwidth. It also speeds up initial page load times. Users have a smoother experience as they interact with the site.
Behind The Scenes: Technical Insight
Lazy loading involves specific techniques and technologies. Here are some key elements:
Component | Description |
---|---|
JavaScript | Handles the loading of resources on scroll. |
HTML Attributes | Uses “data-src” for images instead of “src”. |
Intersection Observer | Detects when elements enter the viewport. |
Lazy loading can be implemented with various libraries. Popular options include:
- LazyLoad.js
- Lozad.js
- Intersection Observer API
Implementing lazy loading can significantly enhance performance. It also leads to a better user experience. Understanding these mechanics is crucial for effective web development.
Benefits Of Implementing Lazy Loading
Lazy loading brings many advantages to websites. It optimizes loading times, reduces server strain, and enhances user satisfaction. Let’s explore these benefits in detail.
Faster Page Load Times
Lazy loading speeds up page loads. It only loads images and content visible to users. This means:
- Less data is downloaded initially.
- Pages appear faster to users.
- Search engines favor quicker sites.
Studies show that faster sites keep visitors longer. Higher engagement leads to better conversion rates.
Reduced Server Load
Lazy loading decreases the demand on servers. It minimizes the number of requests sent at once. Key points include:
- Less bandwidth consumption.
- Fewer resources used during peak times.
- Improved site stability under heavy traffic.
This efficiency allows servers to handle more users simultaneously. It ensures a smoother experience for all visitors.
Improved User Experience
Users enjoy a seamless browsing experience. Lazy loading helps deliver content faster. Benefits include:
- Less frustration waiting for images to load.
- Immediate access to important content.
- Enhanced interaction with the site.
Happy users are more likely to return. They share positive experiences, boosting your site’s reputation.
Lazy Loading And Seo
Lazy loading is a technique that delays loading images and other media. It improves page speed and user experience. Many webmasters wonder how it affects SEO.
Impact On Search Rankings
Lazy loading can have a positive impact on search rankings. Here are some key points:
- Faster Load Times: Pages load quickly, reducing bounce rates.
- Improved User Engagement: Users stay longer on fast-loading pages.
- Better Indexing: Search engines can crawl content efficiently.
However, improper implementation can hurt SEO. Search engines may miss lazy-loaded content. Ensure critical content is visible from the start.
Best Practices For Seo
Follow these best practices to optimize lazy loading for SEO:
- Use Native Lazy Loading: Utilize the
loading="lazy"
attribute for images. - Implement Intersection Observer: Use JavaScript to detect when elements enter the viewport.
- Ensure Accessibility: Provide
alt
tags for images. - Optimize Critical Content: Load important elements before lazy loading other resources.
- Test Performance: Regularly check page speed with tools like Google PageSpeed Insights.
Following these practices will help maintain high SEO standards. Lazy loading can enhance user experience and search visibility.
Types Of Content Suitable For Lazy Loading
Lazy loading helps improve site speed and user experience. Certain types of content benefit the most from this technique. Let’s explore these content types.
Images And Videos
Images and videos often consume a lot of bandwidth. Loading them only when needed saves resources. Here are some key points:
- High-resolution Images: These take longer to load.
- Embedded Videos: Video content can slow down a page.
- Background Images: Load these only when in view.
Lazy loading for images and videos enhances speed. This keeps visitors engaged longer.
Infinite Scroll For Articles
Infinite scroll allows users to browse more content. Lazy loading helps here too. Users see new articles as they scroll down. This method is great for:
- Blogs: Keep readers engaged with endless content.
- News Sites: Offer fresh updates without page reloads.
- Social Media Feeds: Display more posts smoothly.
Lazy loading improves the experience. Users find what they need quickly.
Comments And Social Media Widgets
Comments and social media widgets can slow down pages. Lazy loading helps load these elements only when users interact. Benefits include:
- Reduced Initial Load Time: Users see content faster.
- Less Bandwidth Use: Only load widgets when needed.
- Improved Engagement: Visitors can interact without delays.
This approach keeps your site fast and user-friendly.
Integrating Lazy Loading Into Your Website
Lazy loading improves website speed. It loads images and content only when users need them. This method reduces initial load time and saves bandwidth. Integrating lazy loading can enhance user experience.
Using Javascript For Lazy Loading
Implementing lazy loading with JavaScript is straightforward. You can use the Intersection Observer API. This API detects when an element enters the viewport.
const lazyLoad = () => {
const images = document.querySelectorAll('img[data-src]');
const config = {
rootMargin: '0px',
threshold: 0.1
};
const observer = new IntersectionObserver((entries, observer) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
const img = entry.target;
img.src = img.dataset.src;
img.classList.remove('lazy');
observer.unobserve(img);
}
});
}, config);
images.forEach(image => {
observer.observe(image);
});
};
document.addEventListener('DOMContentLoaded', lazyLoad);
This code snippet initializes lazy loading for images. It sets a threshold for when the images should load.
Plugins And Tools For Implementation
Many plugins simplify the integration of lazy loading. Here are some popular options:
Plugin Name | Description |
---|---|
Lazy Load by WP Rocket | Easy to use. Automatically lazy loads images and videos. |
WP Lazy Loading | Lightweight. Supports images, iframes, and videos. |
Rocket Lazy Load | Fast setup. Lazy loads images without JavaScript. |
Choose a plugin based on your needs. These tools make lazy loading simple and effective. They help improve your site’s performance instantly.
Implementing lazy loading boosts your site’s speed and efficiency. This leads to happier users and better search rankings.
Challenges With Lazy Loading
Lazy loading improves web performance by loading content only when needed. Yet, it has some challenges. Understanding these issues is vital for effective implementation.
Handling Unsupported Browsers
Not all browsers support lazy loading. Some older browsers may ignore lazy loading attributes. This can lead to:
- Images not loading as expected.
- Websites appearing broken or incomplete.
- Poor user experience for some visitors.
To address this, developers can use polyfills. Polyfills add support for lazy loading in unsupported browsers. Here’s a simple example:
Potential Drawbacks And How To Overcome Them
Lazy loading can cause some drawbacks. Here are a few common issues:
Drawback | Solution |
---|---|
SEO Impact | Use proper attributes for search engines. |
Content Visibility | Ensure critical content loads first. |
User Experience | Implement placeholders for content. |
By addressing these challenges, websites can enhance performance without compromising user experience.
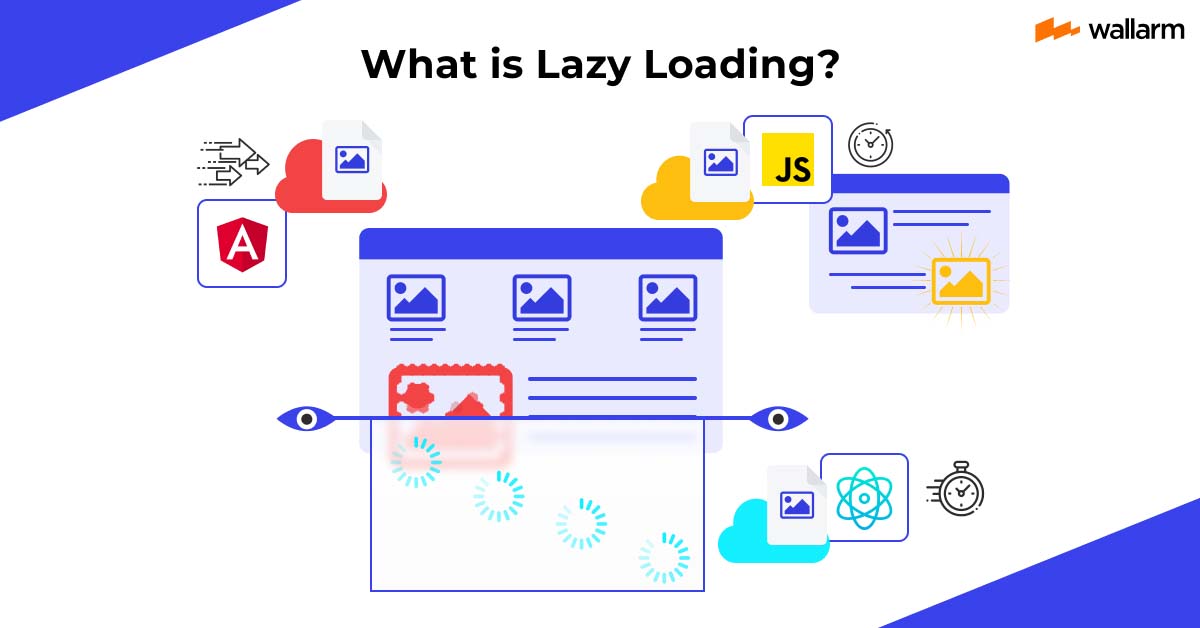
Credit: www.wallarm.com
Lazy Loading In Different Web Development Frameworks
Lazy loading improves web performance by loading resources only when needed. It helps decrease loading times and saves bandwidth. Different web development frameworks implement lazy loading in unique ways. Let’s explore how React, Angular, and Vue.js handle this technique.
Approaches In React
React uses the React.lazy() method. This function allows developers to define components that load on demand.
Here’s a simple example:
const LazyComponent = React.lazy(() => import('./LazyComponent'));
Components wrapped in React.Suspense can show a fallback UI while loading:
Loading...}>
Benefits of lazy loading in React:
- Faster initial loading time
- Improved user experience
- Reduced resource consumption
Lazy Loading In Angular
Angular uses the Router Module for lazy loading. Developers can load modules only when needed, not at the start.
To implement lazy loading, define routes in the routing module:
const routes: Routes = [
{ path: 'lazy', loadChildren: () => import('./lazy/lazy.module').then(m => m.LazyModule) }
];
Angular’s lazy loading benefits include:
- Decreased bundle size
- Faster application startup
- Enhanced performance
Vue.js Techniques
Vue.js uses dynamic imports for lazy loading. The defineAsyncComponent function creates components that load on demand.
Here’s how to use it:
const AsyncComponent = defineAsyncComponent(() => import('./AsyncComponent.vue'));
Vue.js also supports route-level code splitting:
const routes = [
{ path: '/lazy', component: () => import('./Lazy.vue') }
];
Benefits of lazy loading in Vue.js:
- Improved loading speed
- Better resource management
- Enhanced scalability
Case Studies: Real-world Lazy Loading
Lazy loading is changing how websites load content. Many companies use this technique to improve speed and user experience. Here are some real-world examples demonstrating the benefits of lazy loading.
E-commerce Success Stories
E-commerce sites benefit greatly from lazy loading. Faster loading times can lead to increased sales. Here are some success stories:
Company | Before Lazy Loading | After Lazy Loading |
---|---|---|
Shopify | Page load time: 4 seconds | Page load time: 1.5 seconds |
Amazon | Conversion rate: 15% | Conversion rate: 25% |
Etsy | Bounce rate: 40% | Bounce rate: 20% |
These companies saw amazing results. Lazy loading helped boost sales and keep users engaged. Faster sites lead to happy customers.
Impact On Media-heavy Sites
Media-heavy sites rely on images and videos. Lazy loading can significantly improve their performance. Here are some examples:
- Facebook: Uses lazy loading for images in feeds. This keeps the app responsive.
- YouTube: Loads thumbnails only when users scroll. This saves bandwidth and speeds up loading.
- Instagram: Implements lazy loading for posts. This enhances the browsing experience.
These platforms show how lazy loading enhances user experience. Faster loading times keep users engaged. This results in longer visits and more interactions.
Credit: cloudinary.com
Mobile Considerations For Lazy Loading
Lazy loading is crucial for mobile users. It helps pages load faster. This improves user experience significantly. Mobile devices often have slower connections. Lazy loading ensures users see content quickly. Understanding mobile considerations enhances the effectiveness of lazy loading.
Responsive Design And Lazy Loading
Responsive design adapts to different screen sizes. Lazy loading complements responsive design well. Here are key points:
- Images: Load images only when visible.
- Videos: Delay loading until user scrolls.
- Content: Prioritize essential content first.
This approach minimizes data usage. Mobile users appreciate faster loading times. Responsive design combined with lazy loading boosts engagement.
Optimizing For Mobile Users
Mobile users expect quick access to content. Lazy loading helps meet these expectations. Optimize for mobile users by:
- Testing: Regularly test loading speed on various devices.
- Using tools: Utilize tools like Google PageSpeed Insights.
- Monitoring: Keep an eye on user behavior.
Implementing lazy loading can reduce bounce rates. Users stay longer on faster-loading pages. Prioritize mobile optimization for better user satisfaction.
User Experience Design And Lazy Loading
Lazy loading enhances user experience by optimizing content delivery. It loads images and resources only when needed. This speeds up page loading and reduces bounce rates. Users enjoy quicker access to information with less waiting.
Designing For Perception
Perception plays a key role in user experience design. Lazy loading can create a seamless interaction. Here are some ways to design for perception:
- Quick Load Times: Ensure initial content loads fast.
- Smooth Transitions: Use animations for content appearing.
- Visual Hierarchy: Prioritize important elements on the screen.
These strategies help users feel in control. They perceive the site as responsive and friendly.
Placeholder Techniques And Feedback
Using placeholders improves user experience. They give users a visual cue about loading content. Here are some effective techniques:
Technique | Description |
---|---|
Image Placeholders | Display a grey box until the image loads. |
Skeleton Screens | Show a simplified version of the content layout. |
Loading Spinners | Indicate that content is currently loading. |
Feedback helps manage user expectations. Clear signals reduce frustration and keep users engaged.
Advanced Lazy Loading Techniques
Lazy loading improves website performance. Advanced techniques enhance this process. They provide a better user experience. Let’s explore two key methods: Progressive Image Loading and the Intersection Observer API.
Progressive Image Loading
Progressive image loading displays images in stages. This method improves loading speed. Here are key aspects:
- Low-Resolution Images: Start with small, low-quality images.
- High-Resolution Images: Load higher-quality images later.
- Blur-Up Effect: Apply a blur effect to low-res images.
Benefits of progressive image loading:
Benefit | Description |
---|---|
Faster Loading | Users see something quickly. |
Reduced Data Usage | Only load high-quality images when needed. |
Better User Experience | Users stay engaged while images load. |
Intersection Observer Api
The Intersection Observer API monitors visibility. It triggers loading when elements enter the viewport. Here’s how it works:
- Create an instance of the Intersection Observer.
- Set up a callback function for visibility changes.
- Observe the target elements.
Example code for using the Intersection Observer API:
const observer = new IntersectionObserver((entries) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
const img = entry.target;
img.src = img.dataset.src; // Load the image
observer.unobserve(img); // Stop observing
}
});
});
// Observe each image
const images = document.querySelectorAll('img.lazy');
images.forEach(img => {
observer.observe(img);
});
This API helps improve website performance. It only loads elements when they are visible. This reduces initial loading time.
Testing And Measuring Lazy Loading Performance
Testing and measuring lazy loading performance is essential for optimizing your website. It helps ensure faster load times and better user experiences. Accurate measurements can show how well lazy loading works. Tools exist to help analyze these metrics efficiently.
Tools For Performance Testing
Several tools can help test lazy loading performance. Here are some popular options:
- Google PageSpeed Insights: Analyzes page speed and gives suggestions.
- GTmetrix: Provides detailed reports on load times and performance.
- WebPageTest: Offers advanced testing options with various settings.
- Lighthouse: A built-in tool in Chrome for auditing web performance.
Analyzing Load Times And User Engagement
After testing, analyze the data collected. Focus on these key metrics:
Metric | Definition | Importance |
---|---|---|
First Contentful Paint (FCP) | Time taken for the first visible content to load. | Indicates how quickly users see something on the page. |
Time to Interactive (TTI) | Time until the page becomes fully interactive. | Measures how fast users can engage with the content. |
Speed Index | How quickly the contents of a page are visibly populated. | Reflects perceived load speed for users. |
Track user engagement metrics as well. Use tools like Google Analytics to monitor:
- Page views
- Bounce rates
- Session duration
High engagement often correlates with better lazy loading performance. Adjust your strategy based on the data you gather. This will help you improve loading times and user satisfaction.
The Future Of Lazy Loading
Lazy loading continues to evolve. As web technologies advance, new trends emerge. These trends enhance performance and user experience. Businesses focus on faster load times. This leads to higher engagement and lower bounce rates.
Emerging Trends
Several trends shape the future of lazy loading:
- Progressive Web Apps (PWAs): PWAs use lazy loading to improve speed.
- Image Optimization: New formats like WebP enhance image loading.
- Framework Integration: Libraries like React and Vue support lazy loading natively.
- Server-Side Rendering (SSR): SSR combined with lazy loading boosts performance.
Native Browser Support And Standards
Browsers are adopting lazy loading features. This improves user experience across devices. Key standards include:
Browser | Lazy Loading Support | Notes |
---|---|---|
Chrome | Yes | Supports native lazy loading for images and iframes. |
Firefox | Yes | Implementing lazy loading features in upcoming versions. |
Safari | No | Plans to introduce native support soon. |
Edge | Yes | Follows Chrome’s implementation closely. |
These developments create a better browsing experience. As standards evolve, lazy loading will become essential.
Comparing Lazy Loading With Other Optimization Techniques
Lazy loading is a popular technique for improving web performance. It delays loading images and resources until they are needed. Other optimization techniques also enhance speed and efficiency. This section compares lazy loading with minification, CDN utilization, and caching strategies.
Minification And Compression
Minification and compression reduce file sizes. They help load pages faster. Here are the key differences:
Technique | Purpose | Impact |
---|---|---|
Minification | Removes unnecessary characters | Smaller file sizes |
Compression | Reduces file size using algorithms | Faster data transfer |
Lazy loading focuses on loading images only when needed. Minification and compression work on files. All three improve performance but in different ways.
Cdn Utilization
A Content Delivery Network (CDN) distributes content. It stores copies on servers worldwide. This helps users load sites faster. Here’s how it compares:
- Lazy Loading: Loads images only when visible.
- CDN: Reduces distance to server for faster access.
Using a CDN with lazy loading can boost performance. They complement each other effectively.
Caching Strategies
Caching stores copies of web pages. It speeds up access for repeat visitors. Let’s see the key points:
- Lazy Loading: Delays loading images until needed.
- Caching: Saves already loaded pages for quick access.
Lazy loading and caching work together. They both enhance user experience and improve loading times.
Credit: blog.chromium.org
Common Myths About Lazy Loading
Lazy loading improves web performance. Many misunderstand how it works. Let’s clear up some common myths.
Debunking Misconceptions
Several myths surround lazy loading. Here are some common ones:
- Myth 1: Lazy loading slows down the website.
- Myth 2: All images must load at once.
- Myth 3: Lazy loading affects SEO negatively.
Each of these myths is false. Lazy loading actually speeds up initial loading times. Users see content faster. This improves user experience.
Clarifying Expectations
Understanding lazy loading is crucial. Here are some important points:
Expectation | Reality |
---|---|
All images load instantly. | Only visible images load first. |
Lazy loading is hard to implement. | Many simple plugins exist. |
Only affects images. | Can be used for videos and scripts. |
Lazy loading enhances performance. It allows better resource management. Users enjoy a smoother experience.
Accessibility And Lazy Loading
Lazy loading improves web performance. It can also affect accessibility. Users need to access all content easily. This section covers accessibility with lazy loading.
Ensuring Accessible Content
Ensuring content is accessible is crucial. Here are some key practices:
- Proper Alt Text: Use descriptive alt text for images.
- Keyboard Navigation: Ensure users can navigate using a keyboard.
- Screen Reader Compatibility: Test content with screen readers.
- Loading Indicators: Show indicators while content loads.
Lazy loading can hide content from screen readers. Use ARIA attributes to enhance accessibility.
Compliance With Web Standards
Web standards guide accessibility efforts. Follow these guidelines:
Standard | Description |
---|---|
WCAG 2.1 | Web Content Accessibility Guidelines for better usability. |
WAI-ARIA | Web Accessibility Initiative – Accessible Rich Internet Applications. |
HTML5 | Improves semantic structure for better accessibility. |
Compliance ensures that all users can access your content. Regular audits can help maintain accessibility standards.
Best Practices For Implementing Lazy Loading
Lazy loading improves website performance and user experience. Implement it correctly to maximize benefits. Here are some best practices to follow.
Coding Standards
Maintain clean and organized code. This helps prevent errors and ensures smooth functionality. Use the following standards:
- Use HTML5 data attributes for images.
- Employ JavaScript libraries that support lazy loading.
- Follow accessibility guidelines for all users.
- Utilize progressive enhancement for older browsers.
Here’s an example of lazy loading an image:
Use the Intersection Observer API for efficient loading. This method watches elements as they enter the viewport. It reduces unnecessary resource loading.
Testing For Different Scenarios
Testing is vital for ensuring lazy loading works correctly. Test across various devices and browsers. Consider these scenarios:
- Test on mobile and desktop devices.
- Check different screen sizes.
- Ensure images load properly in slow networks.
- Verify behavior with JavaScript disabled.
Use tools like Google Lighthouse to evaluate performance. This helps identify issues and optimize loading times.
Regularly review user feedback. Address any problems users encounter. This keeps the website running smoothly and efficiently.
Troubleshooting Lazy Loading Issues
Lazy loading improves website speed. Sometimes, it can cause issues. Understanding these problems helps you fix them quickly. This section covers common problems and solutions. It also provides expert tips for a smooth deployment.
Common Problems And Solutions
Problem | Solution |
---|---|
Images not loading | Check the image source URL. Ensure it’s correct. |
Content shifts on load | Use fixed dimensions for images. This prevents shifts. |
JavaScript errors | Inspect console for errors. Fix any broken scripts. |
SEO issues | Ensure lazy loaded content is crawlable. Use proper tags. |
Expert Tips For Smooth Deployment
- Test thoroughly: Use various devices and browsers.
- Optimize images: Compress images before lazy loading.
- Use placeholders: Display low-resolution images first.
- Monitor performance: Use tools like Google PageSpeed Insights.
- Implement fallback: Ensure content loads without JavaScript.
- Check browser compatibility.
- Review your lazy loading library’s documentation.
- Adjust loading thresholds to improve user experience.
- Keep your code clean to reduce errors.
By understanding common issues and implementing expert tips, you can enjoy the benefits of lazy loading. A well-optimized site improves user experience and boosts SEO.
Lazy Loading: A Developer’s Perspective
Lazy loading is a technique that optimizes web performance. It helps in loading images and content only when they are needed. This method enhances user experience and reduces loading time. For developers, understanding lazy loading is essential for creating efficient web applications.
Developer Insights
Lazy loading offers several benefits for developers:
- Improved Performance: Loads only necessary elements.
- Reduced Bandwidth: Saves data usage for users.
- Better User Experience: Keeps the interface responsive.
Implementing lazy loading can be simple. Here’s a quick code example using JavaScript:
document.addEventListener("DOMContentLoaded", function() {
const lazyImages = document.querySelectorAll("img.lazy");
const options = {
root: null,
rootMargin: "0px",
threshold: 0.1
};
const lazyLoad = (image) => {
image.src = image.dataset.src;
image.classList.remove("lazy");
};
const observer = new IntersectionObserver((entries, observer) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
lazyLoad(entry.target);
observer.unobserve(entry.target);
}
});
}, options);
lazyImages.forEach(image => {
observer.observe(image);
});
});
This code snippet loads images only when they appear in the viewport. Developers can customize this technique based on their needs.
Community Resources And Support
Developers can find many resources for lazy loading:
Resource | Description |
---|---|
MDN Web Docs | Comprehensive documentation and examples. |
GitHub | Open-source libraries for lazy loading. |
Stack Overflow | Community support for troubleshooting issues. |
Joining forums and communities helps developers share experiences. Engaging with others can lead to new insights. Learning from shared challenges enhances skills.
Frequently Asked Questions
What Is Meant By Lazy Loading?
Lazy loading is a web design technique that delays loading non-essential resources until they are needed. This improves initial load time and reduces bandwidth usage. By loading images and content only when they enter the viewport, websites become faster and more efficient.
Users experience quicker access to essential information.
What Is Lazy Loading Simple Example?
Lazy loading is a technique that delays loading images or content until they are needed. For example, a webpage only loads images as users scroll down. This speeds up initial loading times and improves user experience, especially on mobile devices.
Why Is It Called Lazy Loading?
Lazy loading gets its name because it delays loading non-essential resources until they are needed. This approach reduces initial load time and improves user experience. By loading images or content only when they come into view, it conserves bandwidth and speeds up page performance.
What Is Lazy Loading In Ui?
Lazy loading in UI refers to a design technique that delays loading non-essential resources until they are needed. This improves performance and user experience by reducing initial load times. It’s commonly used for images and other content that users may not immediately see.
What Is Lazy Loading In Web Development?
Lazy loading is a design pattern that delays loading non-essential resources until they are needed.
Why Use Lazy Loading For Images?
It improves page load times by loading images only when they enter the viewport, enhancing user experience.
How Does Lazy Loading Improve Performance?
By reducing initial load time, it conserves bandwidth and speeds up the rendering of the page.
Is Lazy Loading Seo Friendly?
Yes, lazy loading can enhance SEO by improving page speed and reducing bounce rates, benefiting search engine rankings.
Which Elements Can Be Lazy Loaded?
Images, videos, and iframes are commonly lazy loaded to optimize resource loading on web pages.
What Are The Drawbacks Of Lazy Loading?
Potential drawbacks include issues with search engine indexing and delayed content visibility for users.
Conclusion
Lazy loading is a powerful technique that enhances website performance and user experience. By loading images and content only when needed, it reduces initial load times. This approach not only boosts speed but also improves SEO rankings. Embracing lazy loading can lead to happier visitors and better engagement on your site.